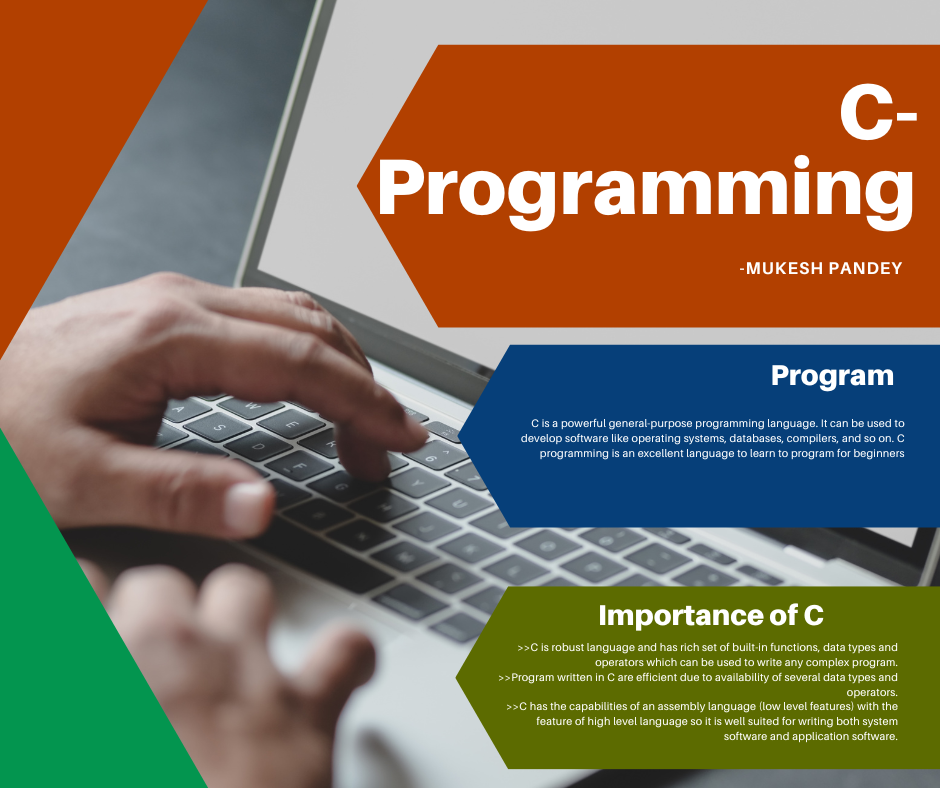
C-Programming Language
Program
- Set of instructions that tells a computer how to perform a particular task.
- They are developed by using programming language.
Software:
- It refers to a program or set of instructions that instructs a computer to perform some tasks.
- They are classified into two classes.
- System Software: Includes OS & various devices software's.
- Application Software: Ms-Word, tally, C Compiler, etc.
Programmer:
- A person who writes a program using a programming language.
- Computer Programming:
- It is a art of developing computer program.
C-Programming
- It is a general- purpose programming language.
- It is used for writing programs.
- It is a high level programming language and easy to learn.
- It is possible to design Operating System, drivers, compilers, etc.
- Almost most of the programming languages like Java, C++, etc. follow C programming syntax.
Features of C-Programming
- Easy to Learn & Understand.
- Machine independent.
- Faster Execution.
- Extensible.
- Reusuable.
Structure of C-Programming
- #include<stdio.h>
- #include<conio.h>
- Int main() {
- Printf(“Hello World \n Hello Ram Singh”);
- return 0;
- }
-------------------------------------------------------------
- #include<stdio.h>
- #include<conio.h> */ No need to write this /*
- Int main()
- {
- Int x=5; // Variable Decleration
- Printf(“Value of x=%d”, x);
- }
----------------------------------------------------------------
Header Files
- It contains definitions of functions and variables, which is imported or used into any C program by using the pre-processor #include statement.
- Header file have extension “.h” which contains C function declaration and macro definition.
- Each header file contains information ( or declarations) for a particular group of functions.
- Like stdio.h header file contains declaration of standard input and output functions available in C which is used for get the input and print the output.
- Similarly, the header file math.h contains declarations of mathematical functions available in C.
C Preprocessor files
- Preprocessor directives are the grammar of C.
- It is used for handling source file and macro definitions in the program.
Comments in C Programming Language
- In C , we can declare comments in two ways:
- 1. Single Line Comment: If we want to place only one line of comment somewhere in program, we can write comment after double forward slashes (//). It does not have ending slashes. For Example: // This is just testing.
- 2. Block Comment/ Multi Line Comment: If we want to place some blocks of comments in a program, we can write comment starting by forward slash asterisk (/*) and write multiple lines of comment and ending with asterisk forward slash (*/). This Comment has starting as well as ending portion. For Example:
- /* This is just for testing
- It takes two values,
- And generates greatest number.
- */
Identifiers, Keywords and Tokens
- Identifier: A name which is given by user it is known as Identifier.
- Keywords: It is a reserved word which have fixed meaning.
- There are 32 keywords in C language.
- Tokens: The basic element recognized by the compiler is known as Tokens.
Basic Data Types in C
- There are 2 types of data types in C. They can be classified as:
1. Primary Data Types
- Char,
- Int,
- Float,
- Double,
- Void
2. Secondary Data Types
-Array,
-Pointer,
-Structure,
-Union,
-Enum
Types of specifier
- The type of data to be printed on standard output is called Specifier. Some of the mostly used specifier are:
- 1. Escape Specifier,
- 2. Format Specifier.
Variable
- A name which can store a value.
- We can re-use that value using of variable.
- For e.g.: Book name is a variable and book price is a value.
Variable Declaration & Initialization
- Type variable name; int. a, b;
- Initialization e.g. :
- a=10; b=20;
- Note: Comma is used to separation.
Variable Declaration rules
- A variable name can have alphabets, digits and underscore.
- E.g. : int a;
- Variable name can start with alphabets and underscore only.
- Variable name cannot be start with digits.
- Whitespace is not allowed in variable name. eg: int student marks;
- A variable name must not be any reserved word or keyword. Eg: int float;
- But we can write int INT;
Practice of Variable
- #include<stdio.h>
- #include<conio.h>
- Int main()
- {
Int a;
a=10;
Printf(“the value of a =%d”, a);
Printf(“a=%d”, a);
Return 0;
}
Define /n also for line break
Constant
- A Constant is a fixed value which cannot be changed during the program execution.
Operators and Expressions
- A operator is a sign or symbol, which performs an operation or evaluation on one or more operands.
- Operands are values or variables dclare within program. For example: 5+10 where ‘+’ sign is an operator and 5 and 10 are operands.
Types of Operator used in C
- Arithmetic Operators,
- Logical Operators,
- Assignment Operators,
- Conditional Operators,
- Bitwise Operators,
- Comma Operators,
- Increment/ Decrement Operators,
- Ternary Operator.
Input/ Output (I/O) Functions
- C provides the following Input/output functions.
- Formatted I/O functions,
- Unformatted I/O functions.
Some of the formatted I/O function are as follows:
S.N. | Function | Description |
1. | Printf() | It is an output function that prints a character or string or numeric values on the screen. |
2. | Scanf() | It is an input function that reads the input from keyboard and different data can be entered like float, char, string into program. |
- Some of the unformatted input output functions are:
S.N. | Function | Description |
1. | getch() | It is an input function which reads only one character at a time i.e. one keystroke without echoing input character on the screen. |
2. | getche() | It is an input function which reads only one character at a time i.e. one keystroke with echoing input character on the screen. |
3. | Putch() | It is an output function that outputs a single character on the screen. |
4. | getchar() | It is an input function to read a character from keyboard. |
5. | Putchar() | It is an output function to print a character on yhe monitor. |
6. | Gets() | It is an input function that reads a single or multiple words from keyboard. |
7. | Puts() | It is an output function that prints a single or multiple words on monitor. |
Control Statement
- It is a way to control a program through control statements.
- There are three types of control statements which control the flow of program.
- Conditional Statement
- Looping Statements
- Jumping Statements.
Conditional Statement
- Conditional Statements:
- Decision Structure:
- It is used to make selection.
- Selection allows us to choose option from two or more options available within the program.
- There are two types of selection statements.
- Two-Way Selection,
- Multi-Way Selection.
- Decision Structure:
Types Conditional Statement
- 1. The Simple If Statement,
- 2. The If-Else Statement,
- 3. The Else If Ladder Statement,
- Nested If Statement,
- Switch Statement.
The Simple IF Statement
Comment
January 11, 2023 10:43Helful
January 11, 2023 10:43Thank you so much
January 11, 2023 10:43Helpful
January 15, 2023 20:54helpful
January 15, 2023 20:54helpful
January 15, 2023 20:54helpful
January 15, 2023 20:54helpful
January 15, 2023 20:54helpful
January 15, 2023 20:54helpful
January 15, 2023 20:54helpful
January 15, 2023 20:54helpful
January 15, 2023 20:54helpful
January 15, 2023 20:54helpful
January 15, 2023 20:54helpful
January 15, 2023 20:54helpful
January 15, 2023 20:54helpful
January 15, 2023 20:54helpful
March 5, 2023 11:00Objective plz
July 19, 2023 04:05Helpful